WordPressから固定ページを取得する
Gatsbyの新しいWordPressインテグレーションを用いれば、既存のWordPressサイトを簡単にヘッドレスWordPressサイトに変換できますが、表示されるのは投稿記事だけです。これに固定ページも表示できるようにする方法のメモです。
gatsby-node.js の変更
- createPages を変更して、固定ページを生成する createWpPages を追加しました。
- また、データを取ってくる関数を getData に改名して、投稿記事に加えて、固定ページを取ってくるようにしました。
exports.createPages = async gatsbyUtilities => {
// Query our posts from the GraphQL server
const { allWpPost, allWpPage } = await getData(gatsbyUtilities)
const posts = allWpPost.edges
const pages = allWpPage.edges
// If there are no posts in WordPress, don't do anything
if (!posts.length) {
return
}
// If there are posts, create pages for them
await createIndividualBlogPostPages({ posts, gatsbyUtilities })
// And a paginated archive
await createBlogPostArchive({ posts, gatsbyUtilities })
await createWpPages({ pages, gatsbyUtilities })
}
- 固定ページを作る関数は次のようにしました。
- 公開されていないページは生成しないようにしています。
const createWpPages = async ({ pages, gatsbyUtilities }) => {
Promise.all(
pages.map(page => {
if (page.node.status === 'publish') {
gatsbyUtilities.actions.createPage({
path: page.node.uri,
component: path.resolve(`./src/templates/page.js`),
context: {
id: page.node.id,
},
})
}
})
)
}
- 投稿記事に追加して、固定ページももってくるようにした関数は次です。
/**
* This function queries Gatsby's GraphQL server and asks for
* All WordPress blog posts. If there are any GraphQL error it throws an error
* Otherwise it will return the posts 🙌
*
* We're passing in the utilities we got from createPages.
* So see https://www.gatsbyjs.com/docs/node-apis/#createPages for more info!
*/
async function getData({ graphql, reporter }) {
const graphqlResult = await graphql(/* GraphQL */ `
query Data {
# Query all WordPress blog posts sorted by date
allWpPost(sort: { fields: [date], order: DESC }) {
edges {
previous {
id
}
# note: this is a GraphQL alias. It renames "node" to "post" for this query
# We're doing this because this "node" is a post! It makes our code more readable further down the line.
post: node {
id
uri
}
next {
id
}
}
}
allWpPage {
edges {
node {
id
status
uri
}
}
}
}
`)
if (graphqlResult.errors) {
reporter.panicOnBuild(
`There was an error loading your blog posts`,
graphqlResult.errors
)
return
}
src\templates\blog-post.js の追加
- gatsby-node.js により固定ページを生成する際に、テンプレートとして用いるのがこちらのファイルです。
import React from "react"
import Layout from "../components/layout"
const PageTemplate = ({ data }) => (
<Layout>
<h1 dangerouslySetInnerHTML={{__html: data.wpPage.title}}/>
<div dangerouslySetInnerHTML={{__html: data.wpPage.content}}/>
</Layout>
)
export default PageTemplate
export const pageQuery = graphql`
query ($id: String!) {
wpPage(id: {eq: $id}) {
id
title
content
featuredImage {
node {
localFile {
childImageSharp {
fluid(quality: 100, maxWidth: 4000) {
...GatsbyImageSharpFluid_withWebp
}
}
}
}
}
}
}
`
結果
- これで、適切なURLを指定すれば、固定ページが表示されます。
- あとはメニューを追加して、そこに到達するためのリンクを付けるだけです。
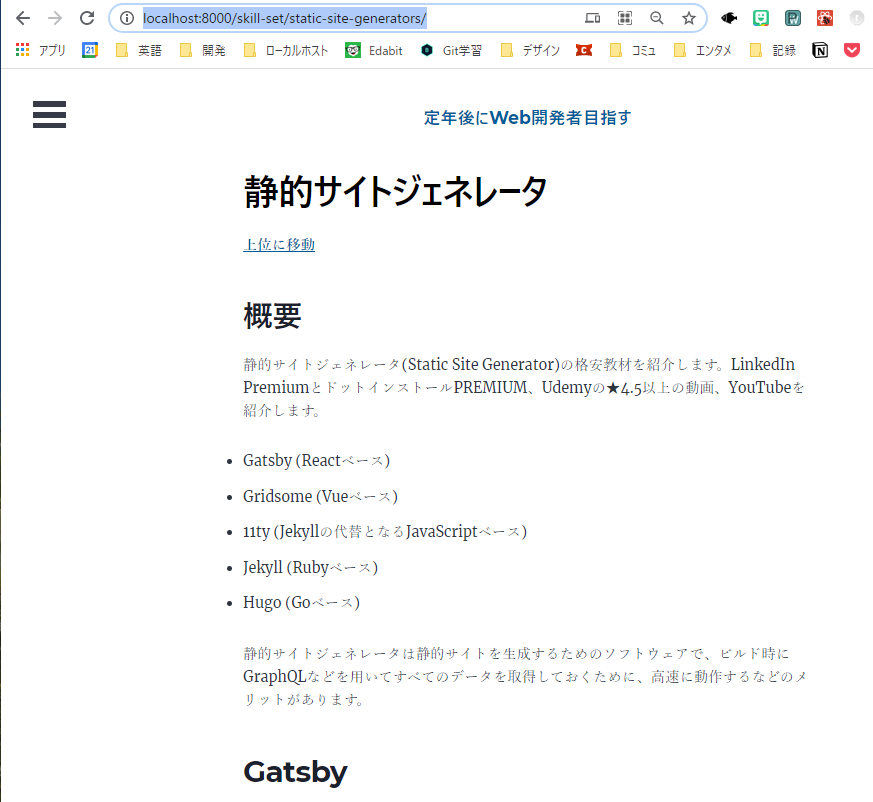
ディスカッション
コメント一覧
まだ、コメントがありません